Unreal
Introduction
Planetary Processing provides a plugin for Unreal Engine 5. The plugin consists of a number of C++ classes for use in connecting players to your game simulation, as well as communicating simulation state to your game client and messages from the client to your simulation.
Pre-requisites
Unreal Engine 5.4+
macOS or Windows, the PP Unreal plugin does not currently support Linux.
Installation
To install the Planetary Processing plugin, you must first download the plugin files from our website, you will need to be logged into your Planetary Processing account to do so, the link is: https://files.planetaryprocessing.io/builds/downloads/artefactid/107/version/latest/dist/dist.tar
Or use a legacy version: 5.4, 5.5.
You'll need then to extract this and move the contents of the lib
directory into a directory called PlanetaryProcessing
in the Plugins
directory at the root level of your project. You'll need to create the Plugins directory if it does not already exist.
You can now enable the Planetary Processing plugin for your Unreal Engine 5 project. You will be required to restart the engine once you enable the plugin.
Classes
PlanetaryProcessing Class
This class is the core of the Planetary Processing plugin. It handles initialization, connection to the server, authentication, message sending, and state updates from the server. This class is intended to be used as a singleton, and instantiated via the Init static function.

Entity Class
This class represents an Entity in the Planetary Processing system. It contains all the data of the corresponding entity on your game server. The Entity Types can be matched up with the EntityActor class using the Entity Map variable.

EntityActor Class
This class extends the Unreal Engine Actor class, spawning an Actor in your game client based on an Entity from your game simulation. You are intended to subclass Entity Actor to create Actors for each of your Entity Types. As an example, a 'Tree' class could be an Entity Actor for a 'tree' Entity Type. Meanwhile a 'Cat' class could be a completely different Entity Actor with a separate mesh component and behaviours.

The EntityActor class is intended to have an Entity associated with it once created, via SetEntity. With this set, the EntityActor class will automatically broadcast OnUpdated and OnRemoved events accordingly, as its associated Entity is either updated or removed.

ChunkActor Class
Use of this class is optional. Similar to the Entity Actor, this class spawns an Actor in your game client. If used, an Actor is spawned at the bottom-left corner of every Chunk in your game simulation.
The main purpose of the Chunk Actor is to store information, such as data about nearby terrain. In most cases the Chunk Actor will not need a mesh, since it cannot move or act.

Chunks only have one type, 'chunk'. A subclass of Chunk Actor must be defined in the Chunk Map using the key 'chunk'. You must also define the Chunk Size integer variable, using the chunk size set in your online game.

The ChunkActor class is intended to have an Chunk associated with it once created, via SetChunk. With this set, the ChunkActor class will automatically broadcast OnUpdated and OnRemoved events accordingly, as its associated Chunk is either updated or goes out of range of a Chunkloader.
MessageData Class
The MessageData class is designed to serve as a flexible container for arbitrary data, akin to JavaScript objects. Its primary purpose is twofold:
Deserialization from JSON: It allows update messages received from the server in JSON format to be efficiently parsed and stored in a way that can be accessed within Unreal Engine. This ensures that the data stored on each Entity (strings, numbers, booleans, arrays and nested objects) can be translated into native Unreal Engine data structures.
Serialization to JSON: Conversely, the class enables the construction of arbitrary data structures which can be serialized and sent to the server as the body of the message in a SendMessage call.
Hence it can be used in Blueprints to both access data on Entity instances and construct messages for SendMessage.
If you want to handle serialization and deserialization yourself, without MessageData, the original JSON of an Entity is available in the DataJSON property of that class. Raw JSON messages can also be sent using the SendJSONMessage function of the PlanetaryProcessing class.

Event Class
The Event Class handles manual server-to-client messaging. Every tick, if a message has been sent from the server using api.client.Message()
, the Unreal custom event OnEventReceived_Event is triggered.
Use this to handle data sent to a specific player client, as opposed to entity data which is sent to all player clients.

Blueprints
The Planetary Processing plugin is packaged with a number of example Blueprints. These serve to show how to carry out some basic functions using the Planetary Processing plugin. These blueprints are PP_ExamplePlayerController, PP_ExampleEntityActor, and the PP_ExampleLoginWidget.
PP_ExamplePlayerController
This blueprint demonstrates how to initialize your Planetary Processing instance and then how to carry out the fundamental processes required to make use of it. These include establishing the connection, initializing entities, maintaining the connection, and processing messages to and from the server.
Authentication
There are two stages to authentication: initializing the connection the game server and joining the validated connection's processing.
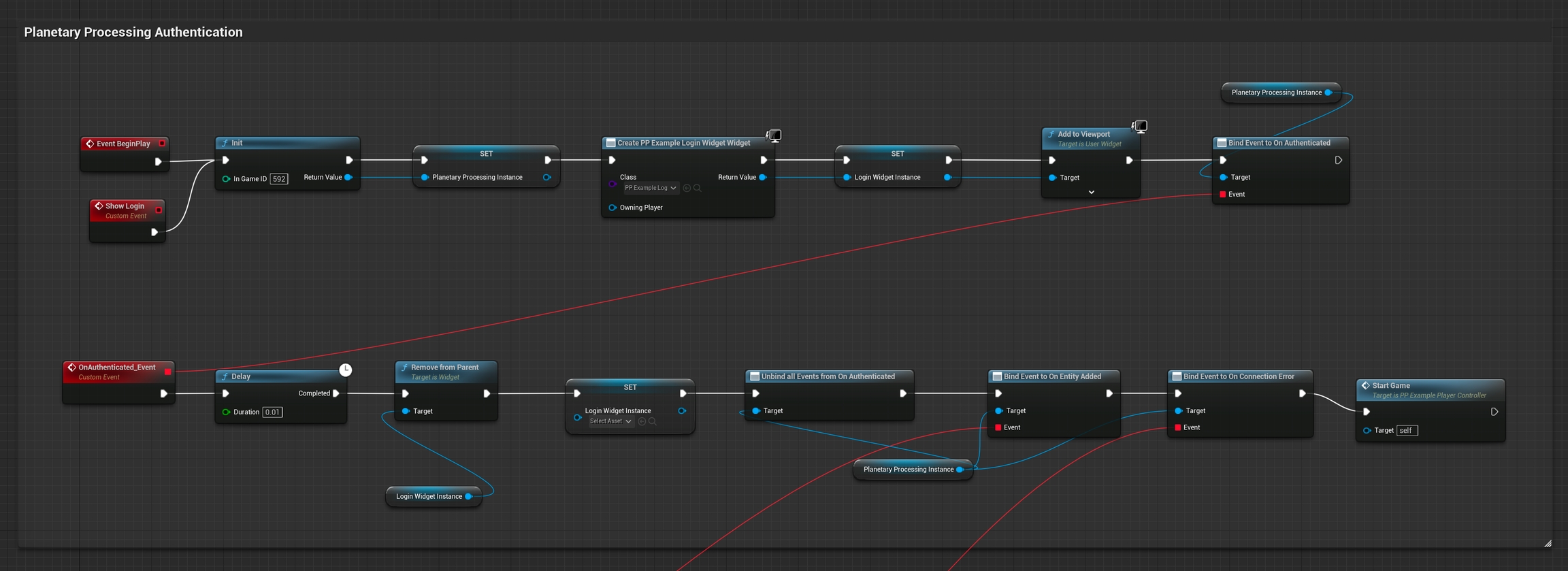
Initializing the connection:
Event BeginPlay/Show Login -> Init -> Set
Initialize the PlanetaryProcessing singleton with a Game ID (Game ID available in the Planetary Processing Panel).
Create PP Example Login Widget Widget -> Set -> Add to Viewport
Create an instance of PP_ExampleLoginWidget and adds it to the viewport.
Planetary Processing Instance -> Bind Event to On Authenticated -> OnAuthenticated_Event
Bind the connection joining processes to the OnAuthenticated event triggered from the PlanetaryProcessing singleton.
Joining a valid connection:
OnAuthenticated_Event -> Delay
A short delay ensures subsequent bindings are handled by the game thread.
Login Widget Instance -> Remove from Parent -> Set
Remove the login widget from the viewport.
Unbind All Events from On Authenticated -> Planetary Processing Instance
Unbind all events from the OnAuthenticated event.
Planetary Processing Instance - > Bind Event to On Entity Added -> Bind Event to On Connection Error
Set up bindings to generate the game server world using the OnEntityAdded and OnConnectionError events from the PlanetaryProcessing singleton.
Start Game -> Start Game (Custom Event)
Continue with game logic, in this case a custom event named StartGame.
Entity Actor Spawning
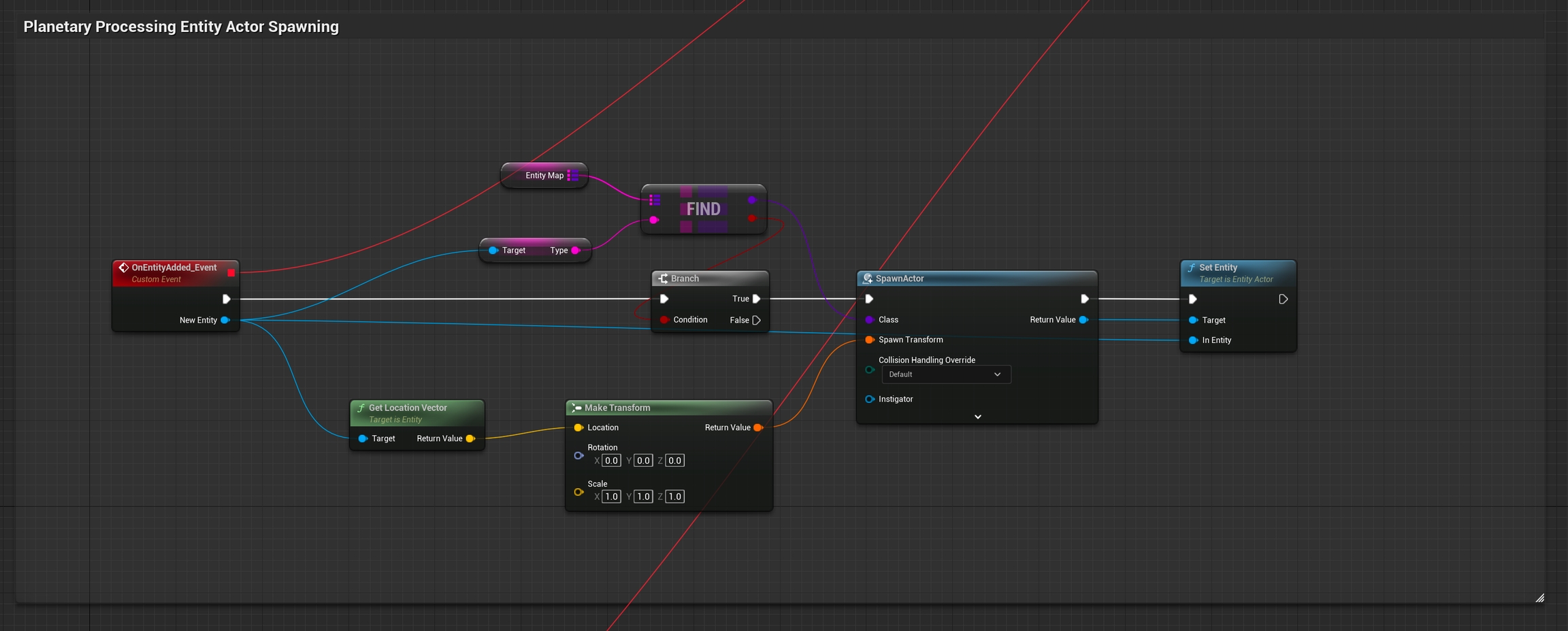
OnEntityAdded_Event -> GetType / Entity Map -> Find
Look up the EntityActor class reference in the EntityMap variable using the Entity Type from the OnEntityAdded_Event.
GetLocationVector -> Make Transform
Use the GetLocationVector function of the added Entity to create a Vector for the initial location of the EntityActor.
Find / Branch / Make Transform -> Spawn Actor
If the Type is valid, spawn an instance of the EntityActor.
OnEntityAdded_Event / Spawn Actor -> Set Entity
Call SetEntity on the EntityActor to associate it with the Entity that we are representing.
If you wish, you can extend the script to remove spawning for the client player entity. This can be done by adding an extra branch to filter entities with the same ID as the Planetary Processing instance's UUID. This only removes the player's own entity from the client, not other players.

Chunk Actor Spawning

OnChunkAdded_Event -> Entity Map -> Find
Look up the ChunkActor class reference in the ChunkMap variable using the key 'chunk'.
GetLocationVector / Chunk Size -> Multiply -> Make Transform
Use the GetLocationVector function of the added Chunk to get its X and Y values. Multiply these by the Chunk Size to create a Vector for the location of the ChunkActor.
Find / Branch / Make Transform -> Spawn Actor
If the 'chunk' key is in use, spawn an instance of the ChunkActor.
OnChunkAdded_Event / Spawn Actor -> Set Chunk
Call SetChunk on the ChunkActor to associate it with the Chunk that we are representing.
Connection Error Handling
If the connection fails, cease processing the world and return to the authentication login.
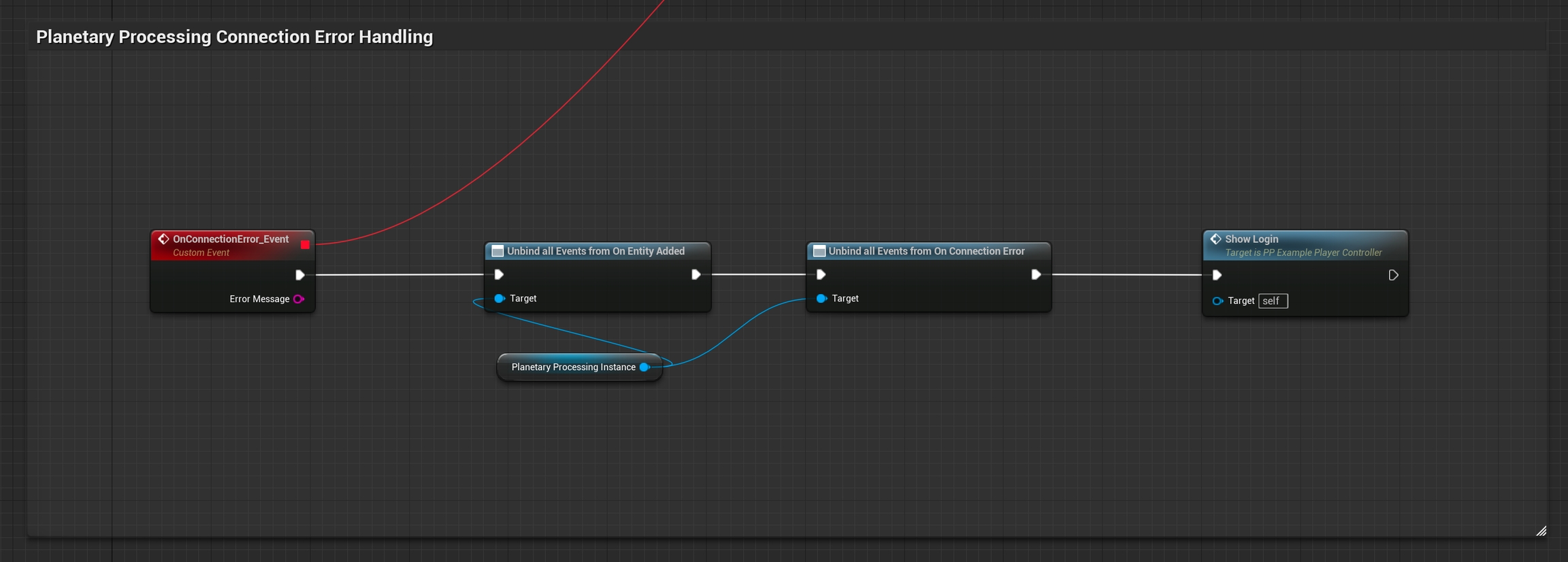
OnConnectionError_Event / Planetary Processing Instance -> Unbind All Events from On Entity Added / Planetary Processing Instance -> Unbind All Events from On Connection Error
Unbinds all events from OnEntityAdded and OnConnectionError.
Show Login -> Show Login Custom Event
Calls a custom event called ShowLogin, which feeds back into the authentication flow above.
Update Entities
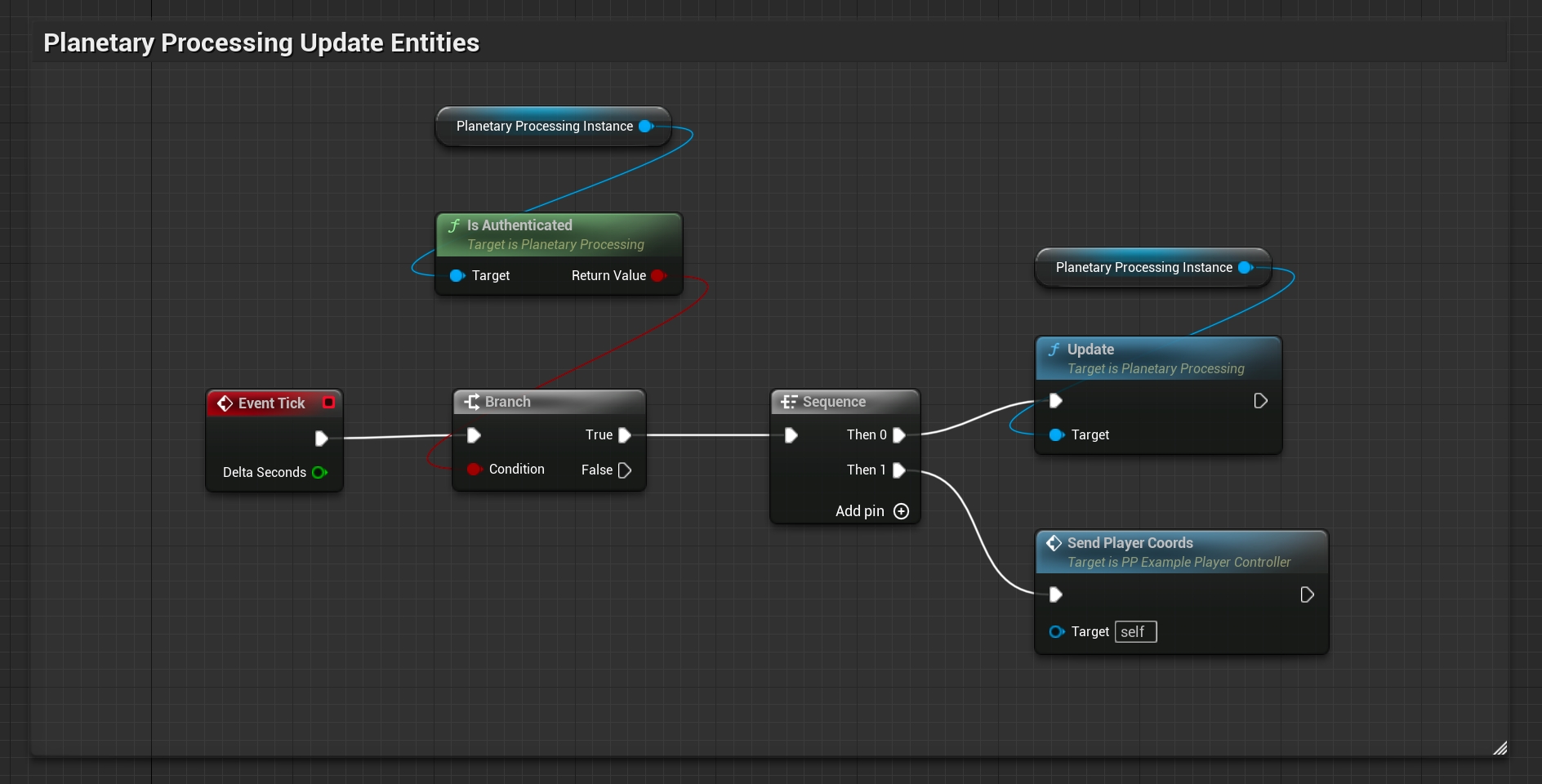
Planetary Processing Instance -> IsAuthenticated / Event Tick -> Branch
On each tick, check if the connection is authenticated by calling IsAuthenticated on the PlanetaryProcessing instance.
Branch -> Sequence / Planetary Processing Instance -> Update -> OnUpdated_Event
If it is authenticated, call the Update function of the PlanetaryProcessing instance to process state changes received.
Sequence -> Send Player Coords -> SendPlayerCoords (Custom Event)
We then also call the SendPlayerCoords custom event.
Send Player Coordinates Message
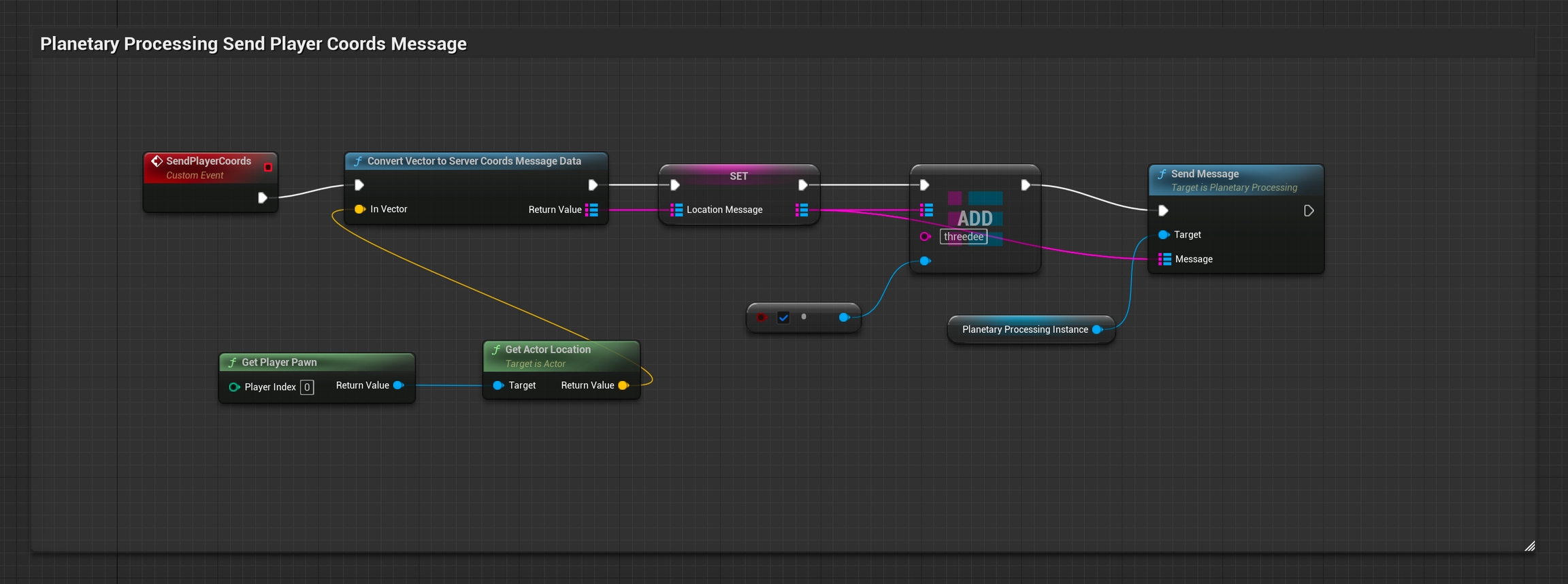
Send Player Coords (Custom Event) -> ...
Process the message on each update tick using the Send Player Coords broadcast.
Get Player Pawn -> Get Actor Location
Get the location of the Player Pawn Actor.
Convert Vector To Server Coords Message Data -> Set
Call the ConvertVectorToServerCoordsMessageData static function from the PlanetaryProcessing class. This gives us a map of strings to MessageData values, with the x, y and z values set based on the player position. ConvertVectorToServerCoordsMessageData alters these xyz values to match the Planetary Processing coordinate system orientation.
Set / To MessageData (bool) -> Add
Uses the implicit conversion of a boolean to MessageData as the value for a new key of threedee in our map.
Add / Planetary Processing Instance / Set -> Send Message
Calls SendMessage on our PlanetaryProcessing instance with our message map as an argument.
Server to Client Event Messaging (example)

OnEventReceived_Event (Custom Event) -> Get Map
Get a message map sent to this player client.
Find -> Get String -> Print String
Use a key to find a specific value in the map, and define what data type that value is expected to be. Then print the value.
PP_ExampleEntityActor
This blueprint shows how to bind to the OnUpdated and OnRemoved events for an EntityActor.
Event Binding
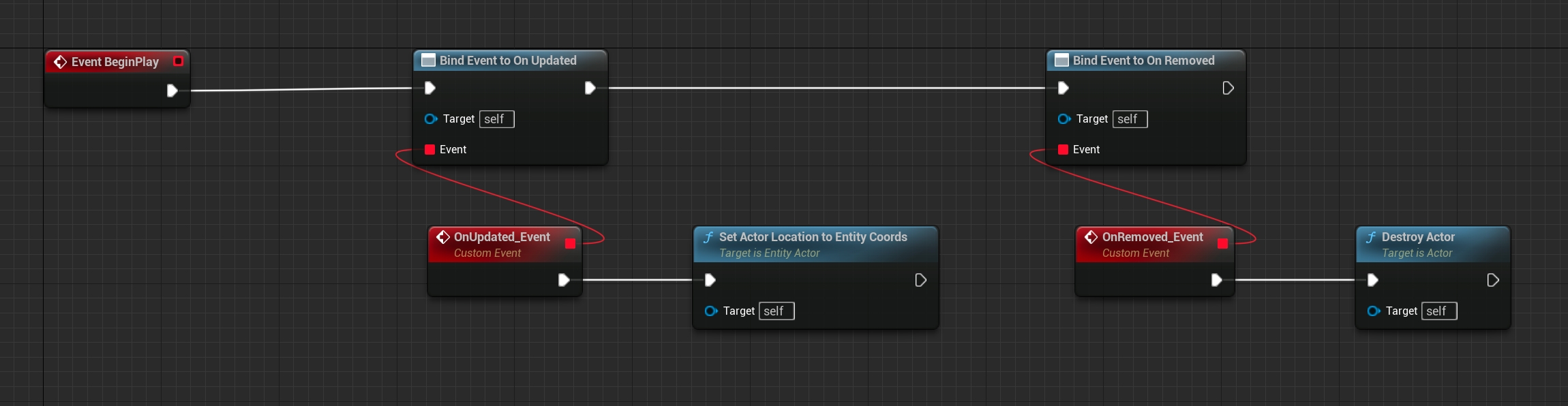
Event BeginPlay -> Bind Event to On Updated -> OnUpdated_Event -> Set Actor Location to Entity Coords
Bind to the OnUpdated event for the EntityActor. Calls SetActorLocationToEntityCoords to update the Actor's location to match the Entity.
Bind Event to On Removed -> OnRemoved_Event -> Destroy Actor
Bind to the OnRemoved event for the EntityActor. Destroys the Actor when this event is broadcast.
For the actor to follow the server-side location, without issue, it must have physics disabled on the clientside.
If you wish to retrieve the location vector of an entity before it is set automatically, you can use the following example. Replace the Print String function with functions of your choice.

PP_ExampleChunkActor
This blueprint shows how to bind to the OnUpdated and OnRemoved events for a ChunkActor.
Event Binding

Bind Event to On Removed -> OnRemoved_Event -> Destroy Actor
Bind to the OnRemoved event for the ChunkActor. Destroys the Actor when this event is broadcast.
PP_ExampleLoginWidget
This is a simple login widget which calls the Connect function and displays an error if the connection fails.
Login Click
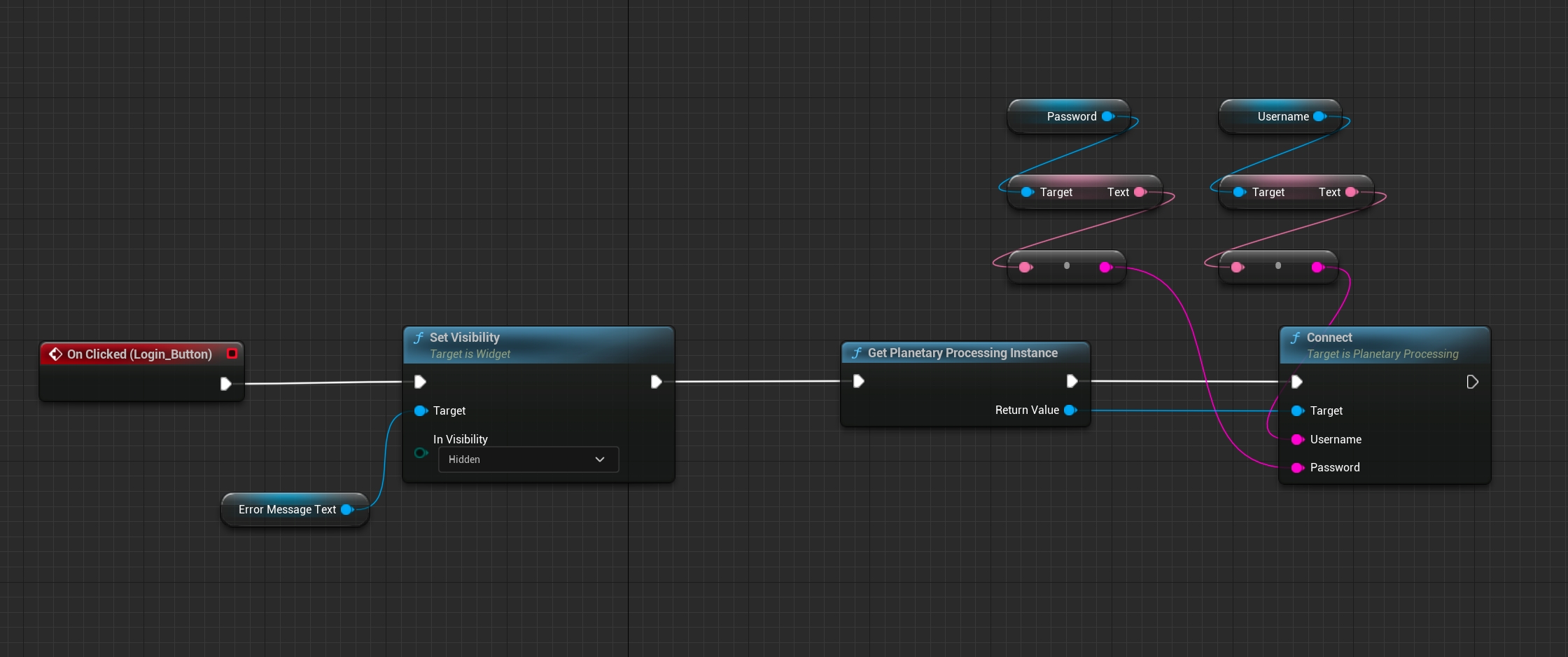
OnClicked (Login_Button) / Error Message Text -> Set Visibility
Hide the error message (effectively resetting the error state of the login form) when clicked.
Username / Password -> GetText -> Get Planetary Processing Instance / To String (Text) -> Connect
Call the Connect function on the PlanetaryProcessing instance with the username and password from the login form.
Error Handling
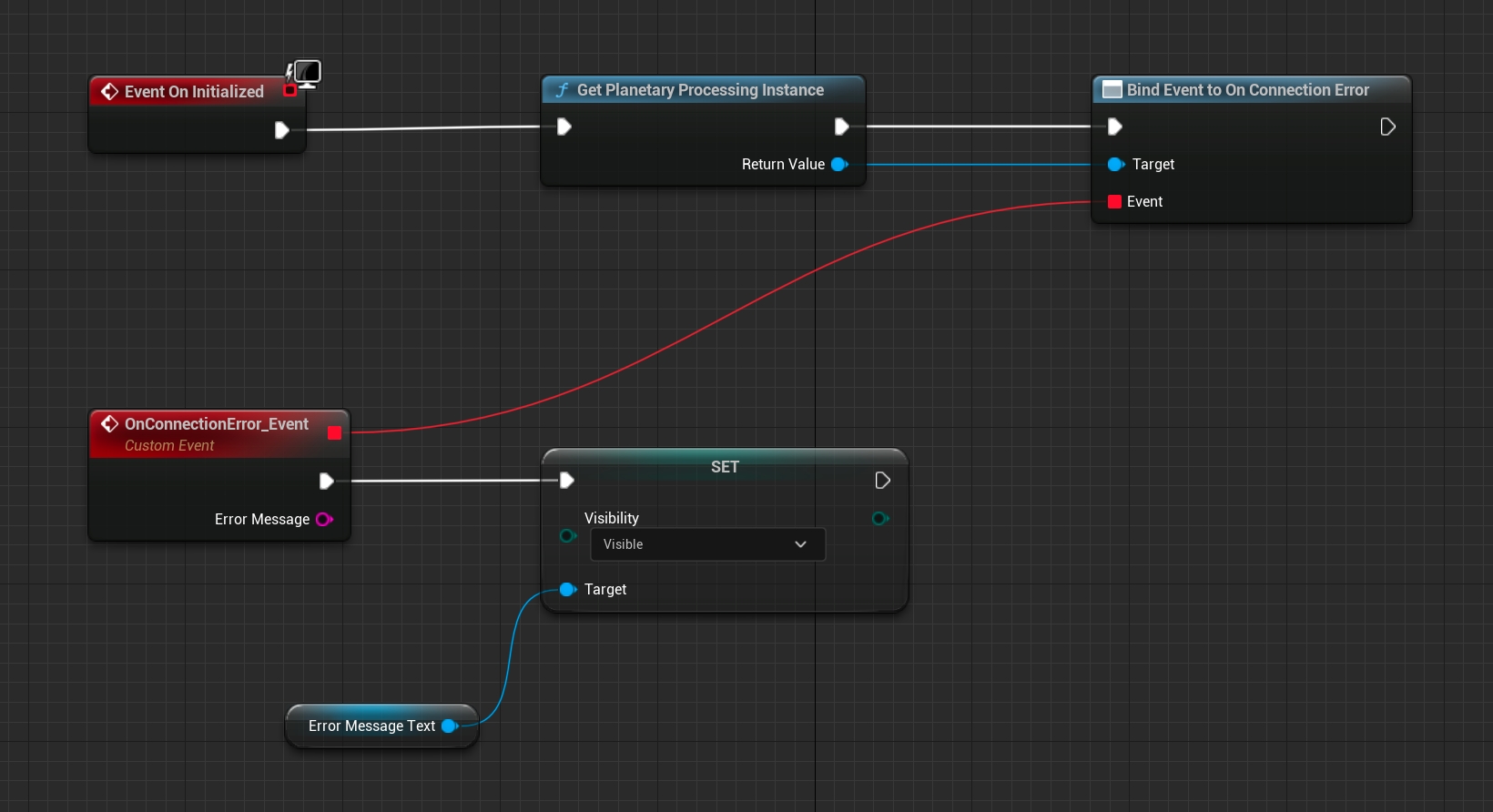
Event On Initialized -> Get Planetary Processing Instance -> Bind Event to On Connection Error -> OnConnectionError_Event
Bind to the OnConnectionError event when the widget is initialized.
OnConnectionError_Event / Error Message Text -> Set
Set the error message to be visible when OnConnectionError is broadcast.
API
PlanetaryProcessing Class
Properties
GameID
int32
ID of the game. Editable in Blueprints.
UUID
FString
Authenticated player's unique identifier. Read-only in Blueprints.
Entities
TMap<FString, UEntity*>
Map of server entities, reflecting the server state as of the latest update. Read-only in Blueprints.
Functions
static Init
int32 InGameID
UPlanetaryProcessing*
Initializes the Planetary Processing singleton with the given game ID. If called again, the existing Planetary Processing instance will be restored to its initial state and returned.
static GetInstance
None
UPlanetaryProcessing*
Gets the singleton instance of the Planetary Processing class.
Connect
const FString& Username, const FString& Password
void
Connects the player to the server with the provided username and password. Broadcasts OnAuthenticated upon successful connection.
Update
None
void
Updates the Entities map by processing state updates that the client has received from the server since the last update. Intended to be called once each game tick such that the Entities map consists of fresh data. Broadcasts OnEntityAdded, OnEntityUpdated and OnEntityRemoved
IsAuthenticated
None
bool
Returns whether the user is authenticated.
IsConnected
None
bool
Returns whether the initial socket connection to the server is active.
SendMessage
const TMap<FString, UMessageData*>& Message
void
Sends a message to the server, using the MessageData format.
SendDirectMessage
const FString TargetUUID,
const TMap<FString, UMessageData*>& Message
void
Sends a message to a specified entity instance on the server, using the MessageData format.
SendJSONMessage
const FString& JsonString
void
Sends a JSON message to the server. This can be used if your data is already in JSON format and as such can be sent directly.
Logout
None
void
Logs out and disconnects the player from the server.
static ConvertVectorToServerCoords
const FVector& InVector
TMap<FString, float>
Converts an Unreal Engine Vector into the coordinate system used in Planetary Processing.
static ConvertVectorToServerCoordsMessageData
const FVector& InVector
TMap<FString, UMessageData*>
Converts an Unreal Engine Vector into MessageData with x, y and z values set in the Planetary Processing coordinate system.
Delegates
OnAuthenticated
None
Fires when player is successfully authenticated.
OnEntityAdded
UEntity* NewEntity
Fires when a new Entity is added to the Entities map. This can be used to spawn a new EntityActor.
OnEntityUpdated
UEntity* UpdatedEntity
Fires when an existing Entity in the Entities map is updated. If an EntityActor has already been spawned based on this Entity, the EntityActor will re-broadcast this as an OnUpdated event.
OnEntityRemoved
const FString& EntityID
Fires when an Entity is removed from the Entities map. If an EntityActor has been spawned based on this Entity, the EntityActor will re-broadcast this as an OnRemoved event.
OnConnectionError
const FString& ErrorMessage
Currently a catch-all for connection related errors, including authentication errors, loss of connection, failure to send messages or failure to parse updates.
Entity Class
Properties
ID
FString
The unique identifier of the entity.
X
double
X coordinate of the entity.
Y
double
Y coordinate of the entity.
Z
double
Z coordinate of the entity.
Data
TMap<FString, UMessageData*>
Additional data of the entity.
DataJSON
FString
JSON representation of the additional entity Data.
Type
FString
Type of the entity.
Functions
GetLocationVector
None
FVector
Converts the Planetary Processing X, Y and Z coordinates of this entity into a Vector in the Unreal Engine coordinate system
EntityActor Class
Properties
Entity
UEntity*
The entity associated with this actor.
OnUpdated
FOnEntityUpdatedEvent
Delegate called when the entity is updated.
OnRemoved
FOnEntityRemovedEvent
Delegate called when the entity is removed.
Functions
SetEntity
UEntity* InEntity
void
Sets the entity for this actor.
SetActorLocationToEntityCoords
None
void
Converts the Planetary Processing X, Y and Z coordinates of the associated Entity into a Vector in the Unreal Engine coordinate system and sets the Actor's location to this Vector
ChunkActor Class
Properties
Chunk
UChunk*
The chunk associated with this actor.
OnUpdated
FOnChunkUpdatedEvent
Delegate called when the chunk is updated.
OnRemoved
FOnChunkRemovedEvent
Delegate called when the chunk is removed.
Functions
SetChunk
UChunk* InChunk
void
Sets the chunk for this actor.
SetActorLocationToChunkCoords
None
void
Converts the Planetary Processing X, Y and Z coordinates of the associated Chunk into a Vector in the Unreal Engine coordinate system and sets the Actor's location to this Vector
MessageData Class
Functions
static ConvertBoolToMessageData
bool Value
UMessageData*
Implicit conversion of a boolean value to a MessageData instance.
static ConvertIntToMessageData
int32 Value
UMessageData*
Implicit conversion of an integer value to a MessageData instance.
static ConvertFloatToMessageData
float Value
UMessageData*
Implicit conversion of a float value to a MessageData instance.
static ConvertStringToMessageData
const FString& Value
UMessageData*
Implicit conversion of a string value to a MessageData instance.
static ConvertArrayToMessageData
const TArray<UMessageData*>& Value
UMessageData*
Implicit conversion of an array of MessageData instances to a MessageData instance.
static ConvertObjectToMessageData
const TMap<FString, UMessageData*>& Value
UMessageData*
Implicit conversion of a map of MessageData instances to a MessageData instance.
GetString
None
FString
Returns the stored string value, if type is String.
GetDouble
None
double
Returns the stored double value, if type is Double.
GetInt
None
int32
Returns the stored integer value, if type is Int.
GetBool
None
bool
Returns the stored boolean value, if type is Bool.
GetObject
None
TMap<FString, UMessageData*>
Returns the stored object value, if type is Object.
GetArray
None
TArray<UMessageData*>
Returns the stored array value, if type is Array.
GetActiveType
None
EVariantType
Returns the active variant type of the message data.
SerializeToJson
None
FString
Serializes the message data to JSON format.
static SerializeMapToJson
const TMap<FString, UMessageData*>& Map
FString
Serializes a map of UMessageData to JSON format.
DeserializeFromJson
const FString& JsonString
void
Deserializes JSON string into message data.
Event Class
Event Data
TMap<FString, UMessageData*>
A manual message received from the server.
PP_ExamplePlayerController
Variables
PlanetaryProcessingInstance
Planetary Processing Object Reference
Reference to the Planetary Processing singleton.
LoginWidgetInstance
PP Example Login Object Reference
Stores a reference to the Login Widget when it is shown.
LocationMessage
TMap<FString: Message Data Object Reference>
A message to be used in a call to SendMessage.
EntityMap
TMap<FString: Entity Actor Class Reference>
Used to map Entity types to the Entity Actor they should spawn. The default values for this variable map both 'cat' and 'tree' to the PP_ExampleEntityActor as a demonstration.
ChunkMap
TMap<FString: Chunk Actor Class Reference>
Used to defined the Chunk Actor for chunks. By default this map is blank, but can be initialised using the key 'chunk'.
ChunkSize
int32
Defines the chunk size on the clientside. Must be the same as on the game server.
PP_ExampleLoginWidget
Variables
Username
UEditableTextBox
A text box widget for entering a username.
Password
UEditableTextBox
A text box widget for entering a password.
Login_Button
UButton
A button widget for triggering the OnClicked event, to connect to the game server.
ErrorMessageText
UTextBlock
Error text displayed, when a connection fails
Last updated